Creating Dynamic Tables in Angular
Cory Rylan
- 3 minutes
Displaying data in tables is a common requirement in our apps. Angular provides declarative features and techniques to create dynamic tables. In this blog post, we will be exploring how to create dynamic tables in Angular by breaking down and explaining a example that leverages these Angular template features.
Component Setup
import 'zone.js/dist/zone';
import { Component } from '@angular/core';
import { CommonModule } from '@angular/common';
import { bootstrapApplication } from '@angular/platform-browser';
@Component({
selector: 'my-app',
standalone: true,
imports: [CommonModule],
template: `...`,
})
export class App {
items = []
}
bootstrapApplication(App);
Starting from the top, we import the necessary modules: zone.js/dist/zone
, @angular/core
, @angular/common
, and @angular/platform-browser
. CommonModule
is imported from @angular/common
to access common Angular directives like ngFor
.
Next, we define a Component using the @Component decorator and leveraging the new standalone component API introduced in Angular 16.
Templating
One of the methods to create DOM quickly from Arrays and Objects the use of the structural directives *ngFor
and the Pipe keyvalue
. These Directives allow for iterating over objects or arrays directly in component templates easily and reflect/update when there are any changes to that data.
@Component({
selector: 'my-app',
standalone: true,
imports: [CommonModule],
template: `
<table>
<tbody>
<tr>
<th *ngFor="let field of items[0] | keyvalue">{{field.key}}</th>
</tr>
<tr *ngFor="let item of items">
<td *ngFor="let field of item | keyvalue">{{field.value}}</td>
</tr>
</tbody>
</table>
`,
})
export class App {
items = [
{ id: 'one', status: 'complete', task: 'build' },
{ id: 'two', status: 'working', task: 'test' },
{ id: 'three', status: 'failed', task: 'deploy' }
]
}
The template is where we define our dynamic table. We utilize HTML table tags (<table>, <tbody>, <tr>, <th>, <td>
) to structure the table and Angular's *ngFor
directive to iterate over the data and display it.
The first <th>
tag uses *ngFor
to loop over the keys of the first item in our items
array, this will create a column header for each key in the item object. The keyvalue
pipe is used to transform the object into an array of key-value pairs, allowing *ngFor
to loop over them.
In the following <tr>
tag, another *ngFor
directive is used to loop over each item
in our items
array. Within each table row, a <td>
tag uses a *ngFor
directive to loop over the fields of the item
object just like our columns, creating a table data cell for each field.
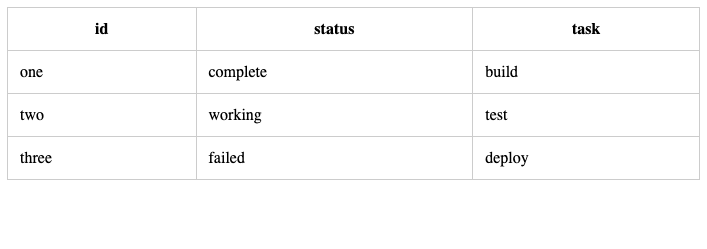
It's important to note that when using the keyvalue
pipe that the default order of the field will be sorted alphabetically as property order is not guarenteed on JavaScript objects.
That wraps up our dive into creating dynamic tables in Angular. This approach allows for great flexibility as the structure of the table adapts to the data, accommodating additional fields or changes in the data structure. Check out the full working demo below!