Understanding Slot Updates with Web Components
Cory Rylan
- 3 minutes
Web Components provide a component model to the Web. Web Components, instead of being a single spec, is a collection of several stand-alone Web technologies. Often Web Components will leverage Shadow DOM features. Shadow DOM is commonly used for CSS encapsulation. However, Shadow DOM has another useful feature called Slots.
The Slot API is a content projection API that allows HTML content from the host application to be rendered into your component template. Common examples of this are things like cards and modals.
<cool-modal>
<p>Stuff that should be rendered in cool modal</p>
</cool-modal>
Here is a minimal example of a Custom Element using the Slot API.
const template = document.createElement('template');
template.innerHTML = `
<div class="inner-template">
<slot></slot>
</div>`;
class XComponent extends HTMLElement {
constructor() {
super();
this.attachShadow({ mode: 'open' });
this.shadowRoot.appendChild(template.content.cloneNode(true));
}
}
customElements.define('x-component', XComponent);
<x-component>
<p>Some Content</p>
</x-component>
The tags' content can be rendered into our template we defined. The browser render the content wherever the <slot>
element is placed. If we look at what the browser renders, we will see something like this:
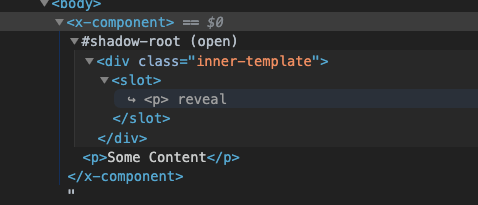
The content is projected and rendered within the template of our component. Often there are use cases, whereas the component author we would like to know about any updates to the content provided by the slot element. We can achieve this by adding an event listener in our component for the slotchange
event.
class XComponent extends HTMLElement {
constructor() {
super();
this.attachShadow({ mode: 'open' });
this.shadowRoot.appendChild(template.content.cloneNode(true));
// get updates when content is updated in the slot
this.shadowRoot.addEventListener('slotchange', event => console.log(event));
}
}
This event will fire whenever any content has changed within the slot. To test this, we can use our component and dynamically update the content to see the event update.
<x-component></x-component>
<script>
setInterval(() => {
// update text content
document.querySelector('x-component').textContent = `${Math.random()}`;
// change the DOM structure
document.querySelector('x-component').innerHTML = `<span>${Math.random()}</span>`;
}, 1000);
</script>
In this example, every one second, we can set the textContent
or the innerHTML
of the component and see the slotchange
event fire within the x-component
.
We can easily render content into our component templates and listen for content updates. But there is one interesting exception to this rule. While the event will happen whenever textContent
or innerHTML
are set, the event will not occur if a textNode
reference is updated dynamically. Let's take a look at an example.
<x-component></x-component>
<script>
const text = document.createTextNode(`${Math.random()}`);
document.querySelector('x-component').appendChild(text);
</script>
Instead of directly setting the textContent
or innerHTML
of our element we create a text node. While not an HTML element, the text node allows us to hold a reference in memory we can update at a later point. So if we go back to our interval, we will see the text change, but the event is no longer triggered.
<x-component></x-component>
<script>
const text = document.createTextNode(`${Math.random()}`);
document.querySelector('x-component').appendChild(text);
setInterval(() => {
// update text node (no slotchange update)
text.data = `${Math.random()}`;
// update text content (triggers slotchange update)
document.querySelector('x-component').textContent = `${Math.random()}`;
// change the DOM structure (triggers slotchange update)
document.querySelector('x-component').innerHTML = `<span>${Math.random()}</span>`;
}, 1000);
</script>
This behavior can be a bit unexpected at first. Many JavaScript frameworks will leverage text nodes to optimize for performance. The short rule to remember is slotchange
only fires when the HTML DOM has changed either by a DOM/Text Node from being added or removed. Check out the full working example below!