Creating Dynamic Tables in Lit
Cory Rylan
- 2 minutes
In this tutorial, we'll dive into creating dynamic tables in Lit, a lightweight, efficient, and powerful library for developing web components. Lit makes it easy to create highly reusable UI components that can be used in any web application. In our example we will create a small HTML table that displays data from a dynamic data source.
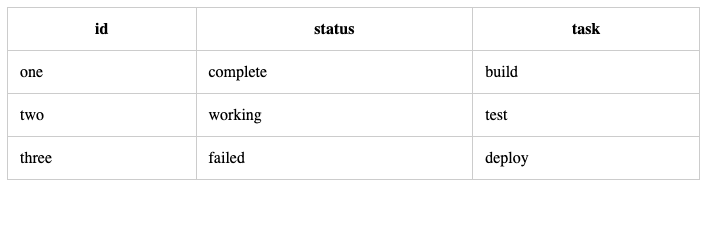
First, import the required modules:
import { html, LitElement } from 'lit';
import { customElement } from 'lit/decorators/custom-element.js';
import { styles } from './element.css.js';
Here we are importing html
and LitElement
from the lit
package. html
is a tagged template function that we will use for creating HTML templates. LitElement
is the base class from which our component will extend. customElement
decorator is used to define a custom element.
Next, we declare a custom element:
@customElement('ui-element')
class Element extends LitElement {
static styles = [styles];
...
}
Using the @customElement
decorator, we create a new custom element called 'ui-element' that extends LitElement
. styles
is an optional property to provide styles for the custom element.
We then define an array of objects, #items
, each object representing a row in the table:
#items = [
{ id: 'one', status: 'complete', task: 'build' },
{ id: 'two', status: 'working', task: 'test' },
{ id: 'three', status: 'failed', task: 'deploy' }
];
Each object has id
, status
, and task
properties which we will use as the columns of our table. Next is the render
function, which is where the dynamic table is created:
render() {
return html`
<table>
<thead>
<tr>
${Object.keys(this.#items[0]).map(i => html`<th>${i}</th>`)}
</tr>
</thead>
<tbody>
${this.#items.map(i => html`
<tr>
${Object.keys(i).map(key => html`<td>${i[key]}</td>`)}
</tr>
`)}
</tbody>
</table>
`;
}
In this function, we create a <table>
with <thead>
and <tbody>
elements. The header row is generated by mapping over the keys of the first item in our #items
array and creating a <th>
element for each key.
The body of the table is generated by mapping over #items
. For each item, we create a <tr>
element. Within this, we map over the keys of the item and create a <td>
element for each key, inserting the corresponding value.
That's it! With this setup, you have a dynamic table in Lit. This table adapts to the structure and contents of the #items
array, allowing flexibility and reusability in your web components. Check out the full working demo in the link below!